Generating a Token
The Victor Platform leverages JSON Web Tokens (JWTs) to identify clients and to ensure the security of requests. In order to generate a JWT, the following requirements must be met:
- API Key must be generated from the client account
- Securely stored private key must be created (this client private key is not recoverable after initial generation, so don't lose it!)
In order to generate your API Key, login to the Client Portal, navigate to My Organization from the Home menu and select the Developer menu item from left-hand options:
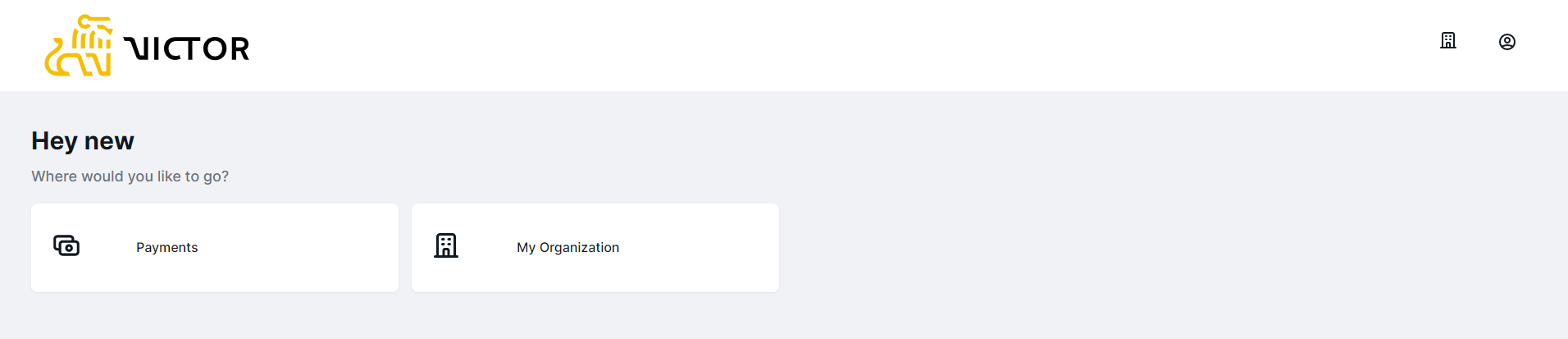
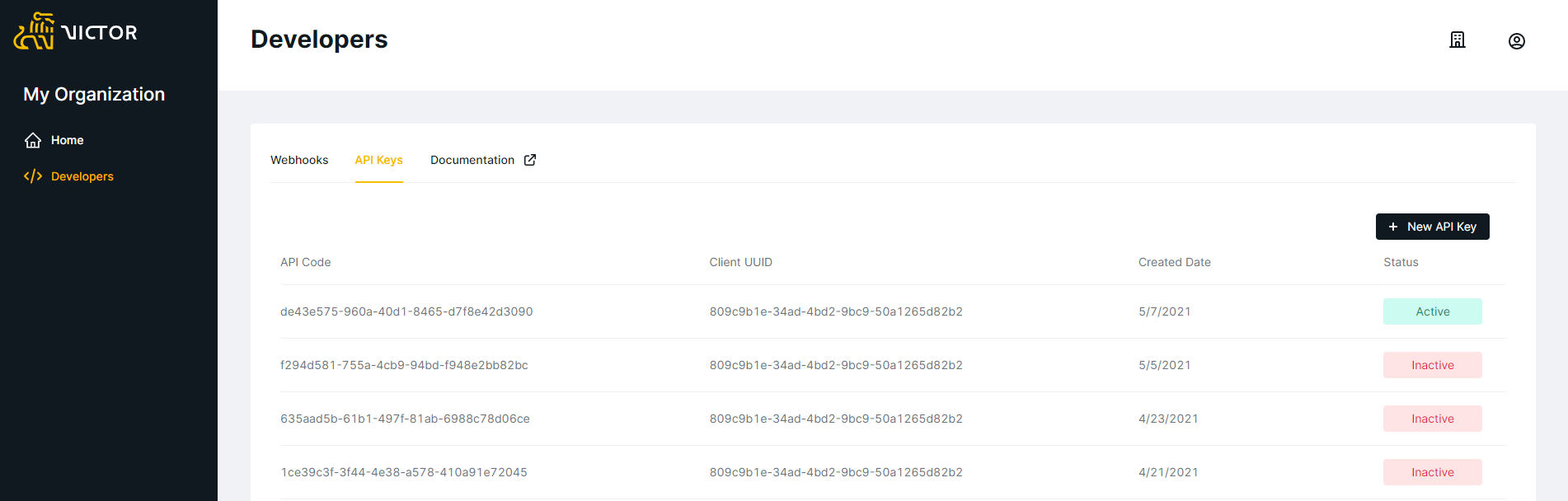
In the Header section of your JWT, use the following:
{
"alg": "RS512",
"typ": "JWT"
}
Use the following details when generating a client JWT:
InfoIssuer: victor-api
Signature Algorithm: RSA-512
Subject: client-api-key
The Payload section of your JWT contains a set of claims. The JWT specification defines seven Registered Claim Names, which are the standard fields commonly used in tokens. For your JWT with Victor, the following claims and values are required:
{
"sub": "65b6f047-c618-485b-a878-833ac3649ec2" (your-api-key),
"iss": "victor-api",
"iat": 1623697929 (issued at timestamp),
"exp": 1623698229 (expiration timestamp)
}
It's important to note that an expiration on the token is required. Ideally, a new token is generated for each request when used in Production.
Heads Up!The maximum expiration time accepted on Production is 300 seconds (
iat
timestamp + 300, or 5 minutes).The maximum expiration time on Staging is 3600 seconds (
iat
timestamp + 3600, or 60 minutes).
You can generate and sign a JWT using multiple methods. A good resource for manually generating and/or verifying JWTs is JWT.io. Below is a code snippet that shows generating and signing a JWT in Java using the Java JWT Library:
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import java.security.KeyFactory;
import java.security.PrivateKey;
import java.security.spec.PKCS8EncodedKeySpec;
import java.util.Base64;
import java.util.Date;
public class JWTGenerator {
public String generateJWT() throws Exception {
Date createdDate = new Date();
Date expirationDate = new Date(createdDate.getTime() + 300);
String privateKeyString = "-----BEGIN PRIVATE KEY-----\n"
+ "Private key\n"
+ "-----END PRIVATE KEY-----";
String keyArray = new String(privateKeyString)
.replace("-----BEGIN PRIVATE KEY-----", "")
.replace("-----END PRIVATE KEY-----", "")
.replaceAll("\\s", "");
byte[] raw = Base64.getDecoder().decode(keyArray);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
PrivateKey privateKey = keyFactory.generatePrivate(new PKCS8EncodedKeySpec(raw));
String jwtToken = Jwts.builder()
.setSubject("API KEY")
.setIssuer("victor-api")
.setIssuedAt(createdDate)
.setExpiration(expirationDate)
.signWith(SignatureAlgorithm.RS512, privateKey)
.compact();
return jwtToken;
}
Once the JWT is generated, it must be included in an Authorization
header within each request, prefixed with the word Token
as shown below:
--header 'Authorization: Token <jwt-string-here>'
Updated 5 months ago